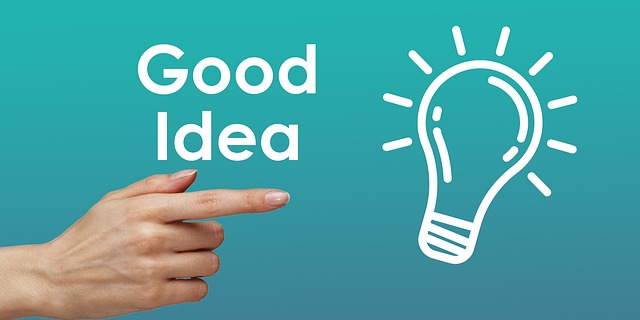
Lets start configuring our Routes using react-router-dom .
Here is the documentation for react-router-dom for reference.
Lets first go to our index.js file in our src folder. Import BrowserRouter, and nest our app in our ReactDOM.render.
//Now import your Router for your routes and wrap your app in it.
import { BrowserRouter } from 'react-router-dom';
Then nest your App Component.
ReactDOM.render(<BrowserRouter><App /></BrowserRouter>, document.getElementById('root'));
Now lets define our routes, go to our routes.js file. Then import React, and also import the Route, and Switch Component from react-router-dom.
//Need to import react becuase the Route itself is a component.
import React from 'react';
//Import the Route, and Switch Comopnent used for defining your routes.
//Route is the route itself which can accept render props, but for the purpose of this tutorial we used the component and path prop.
//Switch responsible for directing to first child that matches thier respective route.
import { Route, Switch } from 'react-router-dom';
Now we will import our Components for each route.
//Now import the components you'll pass as props.
import Home from './components/container/Home/Home';
import About from './components/container/About/About';
import Cart from './components/container/Cart/Cart';
Then we will then just do a export default to our routes, and we do not no need to name them since it is a default export, and name it whatever we want when importing it.
//When dealing with default exports we do not even need to name the export since when it is imported we can name it whatever we want.
export default (
);
Now lets define our routes within the parenthesis of our default export.
We will first use our Switch component. Which will go to the first child matching by it’s route.
<Switch>
//Our Routes
</Switch>
Let’s define our first route. Which will be our home route. Each component has a path which is the path of the route, and the component prop which will take a component to render based on that path. We’ll use the exact keyword before our path so it will go to that exact route. It is useful when defining subroutes within our routes.
{/*Use the exact keyword means it will direct to that exact route, and used primarily when creating sub routes. */}
<Route exact path='/' component={Home} />
Now we can define our routes normally since they are subroutes of the home route. So we will give our Route component a path, and a component prop. Our path will be ‘/about’ and Component will be About.
<Route path='/about' component={About} />
Then we will do the same thing for the Cart Component
<Route path='/cart' component={Cart} />
Great we have our routes now setup. Let start configuring our routes with our Navbar. We will use the this.props.history to redirect the user to that route. Instead of using the window.location. Let’s first import withRouter in our Navbar component in between our react import, and css file import.
//import withRouter so, you can access this.props.history
import { withRouter } from 'react-router-dom';
Now let’s define the method that directs to that route.
//Define your linkFunc so it will redirect to specified route provided via argument.
linkFunc(path) {
this.props.history.push(path);
}
Now for each link we will use the function for the onClick so when the user clicks it will direct them to that route provided as a argument. We will have it in a form of a callback, so we won’t have to bind the method. We will use it for all of them except the login link.
{/* Make sure every link uses linkFunc, except the login link. s*/}
<p className="nav-link" onClick={() => this.linkFunc('/')}>Home</p>
<p className="nav-link" onClick={() => this.linkFunc('/about')}>About</p>
<p className="nav-link" onClick={() => this.linkFunc('/cart')}>Cart</p>
The final step is that we are gonna delete the export default from the class, and instead export the component at the bottom, where we will withRouter HOC which will give it the props of react-router-dom.
Now our links should route to our components set to those routes.
Congratulations! You just setup your routes for your components.
Here is my github for reference.
Leave a Reply